
Just remember that if you go this way you will probably hit a performance ceiling sooner. You can then take advantage of native bitwise operations when generating moves and testing move legality. I found that this implementation was about 13 more efficient, at least in my case.įor example if you wish to describe a valid move for a chess piece, you will need 2 bytes to do so. This helped it save footprint on-chip in comparison to its competitor, which used a 64-bit bitboard. Of course pieces have value, too, but they have an unique id above all. I wrote this article about the same thing: 20130929. This allows much faster move generation and evaluation functions. The main advantage is that you can analyze multiple board positions in a single operation by using bitmasks and shifts. They are not lighter weight as you claim (an 8x8 char array is only 64 bytes), and making a single move is not really faster. Ive recently written this chess engine which implements bitboards in C.Īn even better open source chess engine is StockFish which also implements bitboards but in C. There are other performance advantages too that spring naturally from bitboards representation.įor example you could use lockless hash tables which are an immense advantage when parallelising your search algorithm. The bit corresponding to a pieces location on chess board will be set to 1, all others will be set to 0.įor example, if you have two white rooks on a2 and h1 then the white rooks bitboard will look like this. Typically, the least significant bit will be a1 square, the next b1, then c1 and so on in a row-major fashion. So youll maintain 12 UInt64 variables to represent your chess board: two (one black and one white) for each piece type, namely, pawn, rook, knight, bishop, queen and king.Įvery bit in a UInt64 will correspond to a square on chessboard. It is their material value (bishop and knights are both worth about 3 pawns).īitboards are faster than any array based representation, specially in 64-bit architectures where a bitboard can fit inside a single CPU register. In one bit board you will be storing the position of the rook while in another you will be storing the position of the knight.
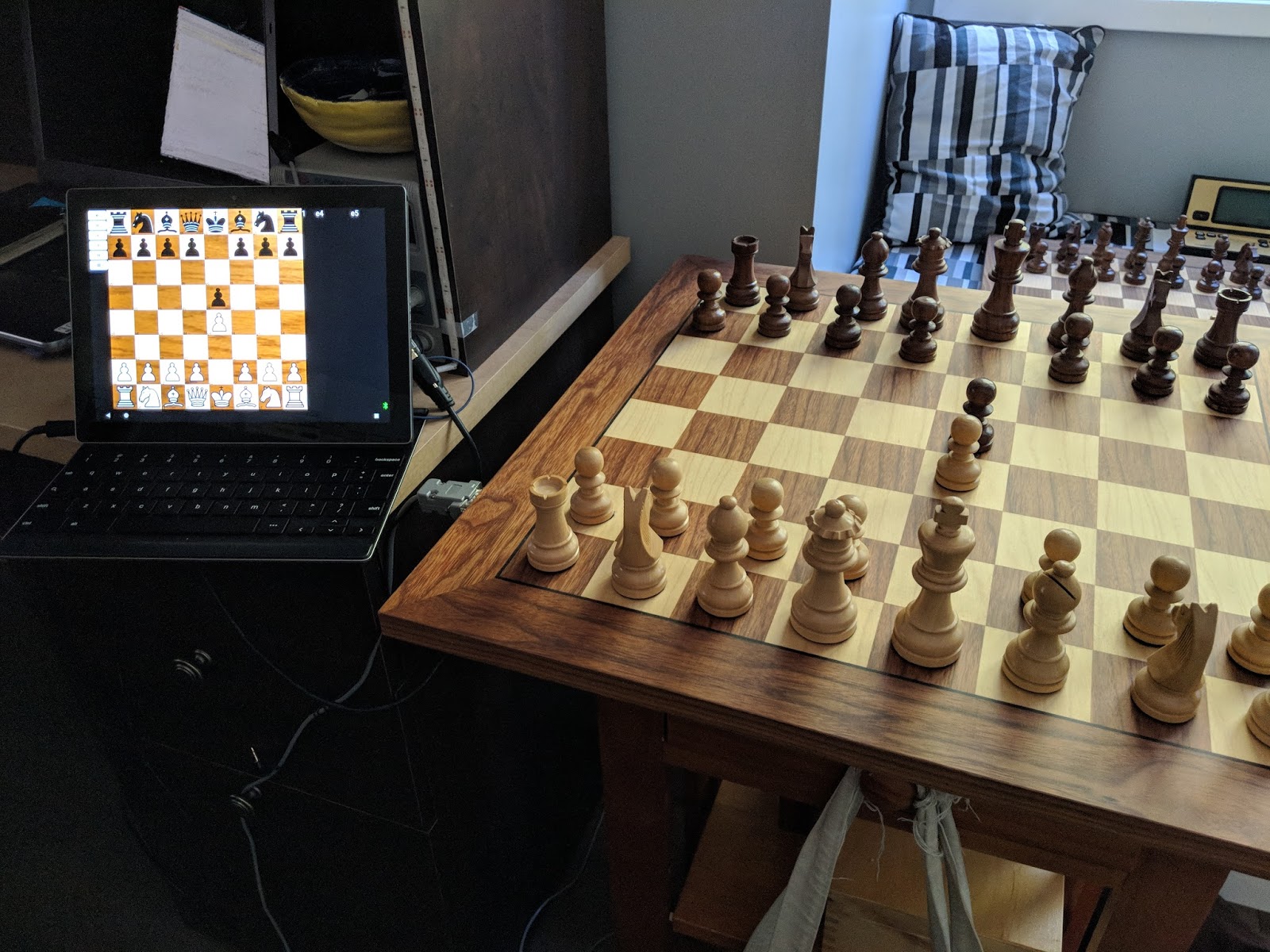
This is a one-dimensional array that includes sentinels around its borders to assist with move generation.
#C PROGRAM FOR CHESS BOARD CODE#
C Program For Chess Board Code Using Cĭelete Replies Reply Reply Reality 5 January 2016 at 16:52 sir do you have a knights tour code using c Reply Delete Replies Reply Anonymous 17 March 2016 at 09:51 can i have the same code in c please Reply Delete Replies Reply Anonymous 30 July 2016 at 02:18 decathlon Reply Delete Replies Reply Unknown at 09:44 can you help me to identify the knights minimum steps to reach from one coordinates to another. Since the algorithm is exponential, optimized input to it can make a huge difference.User entered 3 and 4 which is the initial location of Knight and in Output 0 represent the initial location which is specified by user,and rest numbers representing the possible movement of Knight,so this is example based on this we have to write a program to generate the all possible movement of Knight in chess Board. If we start from the corner, the knight can go to only two points from there. If we start from somewhere middle, the knight can go in 8 different directions. Also, it is always better to start backtracking from any corner of the chessboard. Using the above order, we will get to a vacant position in a few moves. The order in which the knight will move is circular and will be optimum. Important Note: Please avoid changing sequence of above arrays. So, from a position (x, y) in the chessboard, the valid moves are: If the current location is (x, y), we can move to position (x + row, y + col) for 0 <= k <=7 using the following arrays: My goal is not to make a great chess engine. The chess AI algorithms can be improved, more tricks can be used. The AI is a naive minimax implementation. We can find all the possible locations the knight can move to from the given location by using the array that stores the knight movement’s relative position from any location. No castling or en passant, pawn gets promoted to queen. We know that a knight can move in 8 possible directions from a given square, as illustrated in the following figure:
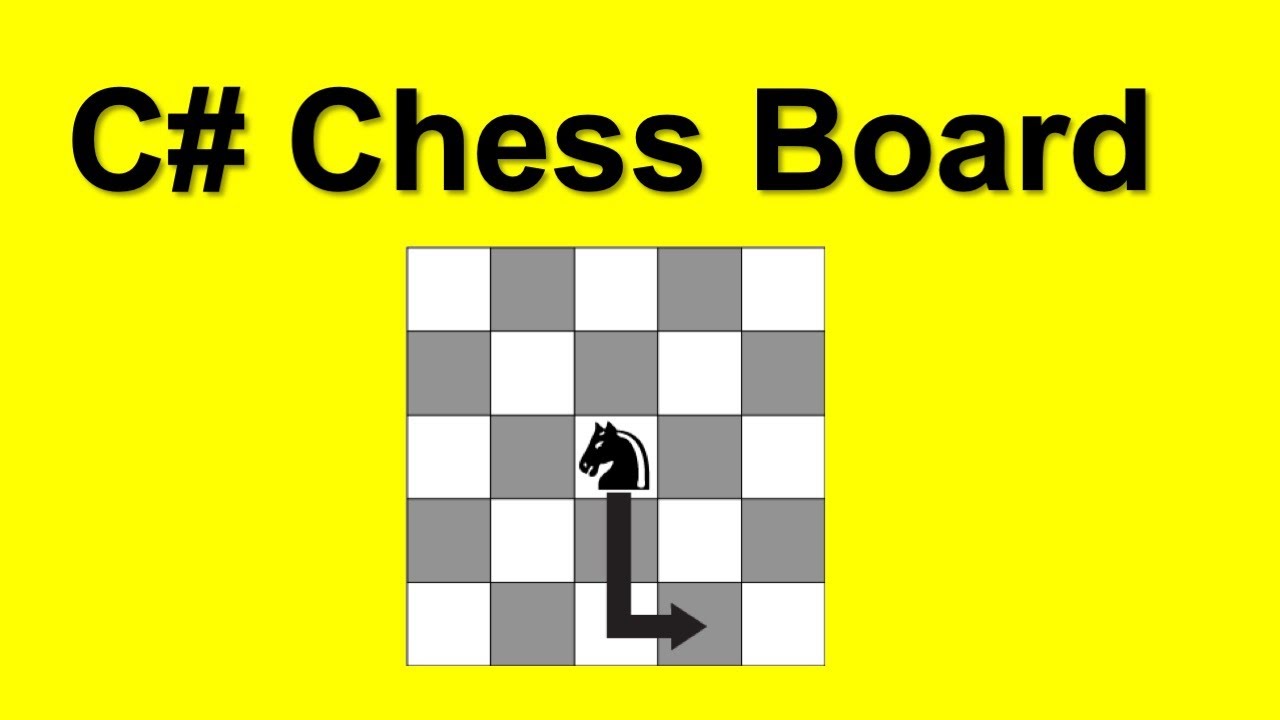
To make sure that the path is simple and doesn’t contain any cycles, keep track of squares involved in the current path in a chessboard, and before exploring any square, ignore the square if it is already covered in the current path. If the current path doesn’t reach the destination or explored all possible routes from the current square, backtrack.
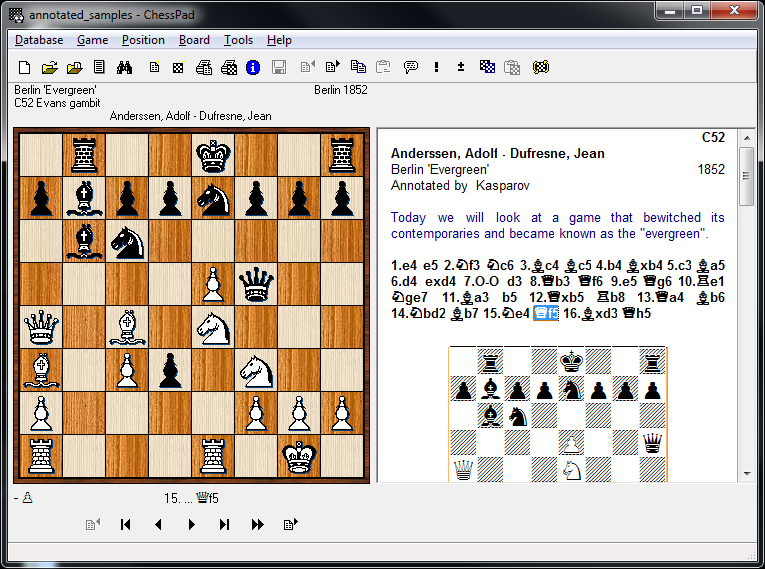
We start from the given source square in the chessboard and recursively explore all eight paths possible to check if they lead to the solution or not. We can easily achieve this with the help of backtracking. The knight should search for a path from the starting position until it visits every square or exhausts all possibilities. Chess Knight Problem | Find the shortest path from source to destination
